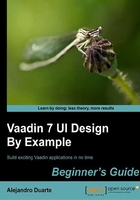
Time for action – binding data to properties
There's no better way to learn than practice. Follow these steps to see how binding works in real life:
- Create a new project named binding using your IDE. A Vaadin one, of course.
- Don't hesitate and delete all the content in the generated
BindingUI
class. - Create a new local instance of
TextField
and turn immediate mode on:@Override protected void init(VaadinRequest request) { TextField textField = new TextField("Data"); textField.setImmediate(true); }
- Create a new local instance of
Label
:Label label = new Label();
- Add the
TextField
andLabel
components to a newVerticalLayout
and set it to be the content of the UI:VerticalLayout layout = new VerticalLayout(); layout.addComponent(textField); layout.addComponent(label); setContent(layout);
- Nothing new so far, so create a new instance of the
ObjectProperty
class:ObjectProperty<String> property = new ObjectProperty<String>("the value");
- Wow, that was new. More newness, bind the property to the UI components:
textField.setPropertyDataSource(property); label.setPropertyDataSource(property);
- Deploy and run the application. Try changing the value in the
textField
component. While testing the application, make sure you click out of thetextField
or press Tab after changing its value, so the change will be sent to the server.
What just happened?
We bound two UI components to a single data source. One of the components (TextField
) is capable of changing the data source (ObjectProperty
) while the other just displays the data in the data source. Here is a screenshot:

If you change the value in the TextField
component, it will in turn change the data source, causing the Label
component to display the new value. textField
and label
are both connected to the same data source via the ObjectProperty
instance:

This is useful because we can easily attach a single data source to multiple views (UI components).
What is property
anyway? It is an object containing a value of a certain type. In the previous example, the type was String
. The Property
class is an interface, and Vaadin provides several implementations for this interface. We are using the ObjectProperty
implementation that allows us to wrap any Java object into a property.
The Property
interface has some methods to get and set the value, to get and set the read only state, and to get the type of the stored value. This is a graphical depiction of a property:

Items
Items are a way to group properties. Before grouping the properties each one receives an ID, the property ID (or PID):

Think of a Java object. It has several properties each one with its own name. For example:
class User { String login = "bill"; String password = "pass"; Boolean enabled = true; }
We can model this data abstraction using properties and items:

Items are used in components that deal with more complex data than labels or text fields. For example, Vaadin includes a FormGroup
class that can be bound to an item.
Containers
Last, but not least, a container is a way to group items. And again, before grouping the items, each one receives an ID, the item ID (or IID):

Two common Vaadin components that can be bound to containers are Table
and Tree
.