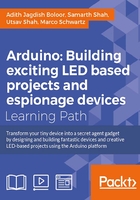
Chapter 5. Burglar Alarm – Part 2
This is part 2 (and the final part) of the burglar alarm series. So far, we have configured the camera, the Bluetooth, and Python.
In this chapter, we will be going through the following topics:
- Obtaining and processing the image of the intruder
- Uploading the image to a convenient website
- Sending the URL to your smart phone
So, shall we get right to it?
Dealing with the image
As discussed before, when Arduino sends a message to Python, it is going to take a snapshot using the camera and save it to the computer. What do we do with it, then? How do we upload it to a file sharing platform? There are several ways to do this, but in this chapter, we will be using Imgur. Yes, the same Imgur that you have been using, knowingly or unknowingly, on Reddit or 9gag.
Go to http://imgur.com/ and sign up for a new account.
Once you have verified your account, go to https://api.imgur.com/oauth2/addclient to add a new application so that Imgur permits you to post images using a script. Use the following information:
- Application name: Arduino burglar alarm
- Authorization type: OAuth 2 authorization without callback URL
- Email: <your email>
- Description: Using Arduino to catch the cookie thieves
Now proceed, and you will get a page like this:

Imgur will give you the Client ID and Client secret. Save them in a safe location as we will need them later.
Let us try testing the automatic image uploading functionality. However, before that, we need to install the Imgur library for Python. To do this, firstly, we need to install a simple Python library installer called pip
, which is used to download other libraries. Yeah! Welcome to Python!
Go to you could just use get-pip.py
that is in the Useful Files
folder that came with this chapter. Save it or copy it to C:\Python27
. Go to your C
directory and Shift + right-click on Python27
. Then, select Open command window here:

Type python get-pip.py
, hit Enter, and let it install pip to your computer.
Navigate to C:\Python27
and Shift+ right-click on Scripts. Open the command window and type pip install pyimgur
in that command window to install the Imgur library. Your terminal window will look like this:

Let's test this newly installed library. Open Eclipse and create a new file called imgur_test.py
, and paste the following or simply load the imgur_test.py
file from the code attached to this chapter:
import pyimgur # imgur library import urllib # url fetching default library import time # time library to add delay like in Arduino CLIENT_ID = "<your client ID>" # imgur client ID # retrieve the snapshot from the camera and save it as an image in the chosen directory urllib.urlretrieve("http://<your ip : port>/snapshot.cgi?user=admin&pwd=password", "mug_shot.jpg") time.sleep(2) PATH = "C:\\<your python project name>\\mug_shot.jpg" # location where the image is saved im = pyimgur.Imgur(CLIENT_ID) # authenticates into the imgur platform using the client ID uploaded_image = im.upload_image(PATH, title="Uploaded with PyImgur") # uploads the image privately print(uploaded_image.link) # fetches the link of the image URL
Change <your client ID>
to the ID that you saved when you created an application on Imgur; change <your ip : your port>
to your camera URL; change <your python project name>
to the folder where all your python codes are saved. For example, if you look at your Eclipse IDE:

My project name is helloworld
, and in the C
drive I have a folder called helloworld
where all the Python files are saved. So, set your PATH
to correspond to that directory. For me, it will be C:\\helloworld\\mug_shot.jpg
where mug_shot.jpg
is the name of the saved file.
Once you have changed everything and ensured that your camera is on the wireless network, run the code. Run it using Python as you did in the helloworld.py
example in the previous chapter and you should get a result with an Imgur link:

Copy and paste this link in a new browser window and you should have something like this:

Try refreshing the page and see what happens. Nothing! Exactly! This is why we took all the trouble of saving the image and then uploading it to a file sharing server. Because once the snapshot has been taken, it will not change.
Be mindful while using this method to upload images to Imgur. Do not abuse the system to upload too many images, because then your account will be banned.
Now, it is time to deal with sending a notification to your smart device.
Sending a notification to a smart device
There are many ways a notification can be sent to your smart device (e-mail, SMS, or via an app). During the course of writing this chapter, I realized that an e-mail is not the most efficient way to alert the user of an emergency (a burglar in this case), and there is no single global SMS notification service that can be used by people from different parts of the world. Hence, we are going to use yet another really powerful push messaging app called Pushover.
It works by communicating over the Internet, and conveniently there is a Python library associated with it that we will use to send notifications to the Pushover app on our smart device.

Go to is sufficient for completing this chapter. If you like the software, you can go ahead and purchase it, as you can use it for future projects.
Once you have verified your e-mail ID, look up Pushover on the iTunes App store (https://itunes.apple.com/en/app/pushover-notifications/id506088175?mt=8) or on the Google Playstore (https://play.google.com/store/apps/details?id=net.superblock.pushover&hl=en) and download the app. Log in with the same credentials. It is important to allow push notifications for this app, because that is going to be its sole purpose.
Now, when you go back to devices listed, as shown in the following:

Note down your user key as we will need it while we create a script. For now, let us test the app. Under Send a notification, fill in the following:

Now, hit the Send Notification button. Wait for a couple of seconds and you should see a message that pops up on your smart device with the title Hello World:

Pretty neat, huh?
Now, go ahead and register a new application on the Pushover website:
- Fill in the details as you wish:
- Then, click on Create Application and you'll get the following page:
- Note down your API Token/Key.
- Go to
C:\Python27
and Shift + right-click on Scripts; open a command window and type the following:pip install python-pushover
- Hit Enter. This will install the
python-pushover
library for you. - Open Eclipse and create a new script, and call it
pushover_test.py
. Copy the following code into it, or load thepushover_test.py
file that came with this chapter:from pushover import init, Client init("<token>") client = Client("<user-key>").send_message("Hello!", title="Hello")
- As you did for the Imgur tutorial, change
<token>
to your API Token/Key. Also, change<user-key>
to your user key that is available when you sign in to your account at https://pushover.net/.
Run the script. Wait for a few seconds and you should get a similar notification as you got before. Fascinating stuff, if I say so myself.
Finally, we have finished working with and understanding the elements that will go into creating the entire compound. We are now going to put them all together.
Putting the pieces together
Go ahead and create this circuit for Arduino:

The connections are as follows:
- PIR → Arduino
- GND → GND
- OUT → D02
- VCC → 5V
- HC-06 → Arduino
- VCC → 3.3V
- GND → GND
- TXD → D10
- RXD → D11
Don't get mad, but there is one last library that you need to install in order to allow Arduino to communicate with Python: pySerial
. Go to install it just like you install any other software. Then, we are ready.
Open Arduino and load the alarm_bluetooth.ino
file that came with this chapter. It is recommended that you have the most up-to-date Arduino software before proceeding:
#include <SoftwareSerial.h> // serial library used for communication SoftwareSerial burg_alarm(10, 11); // communicates via TX, RX at 10, 11 respectively int ledPin = 13; // in built LED to show status changes int pirPIN = 2; // signal of the PIR sensor goes to pin 2 int pirState = LOW; // initiate the PIR status to LOW (no motion) int pirVal = 0; // variable for storing the PIR status void setup() { burg_alarm.begin(9600); // communication begins on baud 9600 pinMode(ledPin, OUTPUT); // sets the LED pin as output delay(5000); // waits 5 seconds for motion to die down } void loop(){ pirVal = digitalRead(pirPIN); // read input from the sensor if (pirVal == HIGH) { // if input is HIGH (motion detected) digitalWrite(ledPin, HIGH); // turn LED ON delay(150); // small delay if (pirState == LOW) { // checks if the PIR state is LOW while the input is HIGH // this means, there wasn't motion before, but there is something happening now Serial.println("Motion detected!"); // prints out an Alert burg_alarm.println('1'); // sends out '1' for when motion is detected via Bluetooth to python pirState = HIGH; // sets the pirState to HIGH (motion detected) } } else { // no motion detected digitalWrite(ledPin, LOW); // turn LED OFF delay(300); // small delay if (pirState == HIGH){ // if there was motion, but isn't any now Serial.println("Motion ended!"); burg_alarm.println('0'); // sends a '0' when the motion has ended pirState = LOW; // sets the state to LOW (no motion) } } }
Make sure you unplug the TX and RX wires before uploading the code and then attach them back. Now, unplug the Arduino while we create a Python script.
Open Eclipse and load the alarm_bluetooth.py
file, or just copy the following:
import serial import pyimgur import urllib import time from pushover import init, Client print("Burglar Alarm Program Initializing") init("< your push overtoken>") CLIENT_ID = "<your client ID>" PATH = "C:\\<your python folder>\\mug_shot.jpg" im = pyimgur.Imgur(CLIENT_ID) mug_shot_ctr = 0 serial_status = 0 camera_status = 0 try: print("\nAttempting to connect to Bluetooth module...") ser = serial.Serial('COM36', 9600) #Tried with and without the last 3 parameters, and also at 1Mbps, same happens. time.sleep(3) serial_status = 1 print('Bluetooth connection successful!') except: print('Bluetooth Connection Error! \nPlease check if bluetooth is connected.') mug_shot_ctr = 4 try: print("\nChecking IP Camera Status...") urllib.urlretrieve("http://<your ip>/snapshot.cgi?user=admin&pwd=<your password>", "mug_shot.jpg") time.sleep(2) print("Camera Status OK") camera_status = 1 except: print("Camera not connected!\nPlease check if camera is connected.") mug_shot_ctr = 4 if((serial_status==1)&(camera_status==1)): print("\nBurglar Alarm armed!") while mug_shot_ctr < 3: line = ser.readline() if(line[0]=='1'): print('\nMotion Detected!') print('Capturing Mug Shot') urllib.urlretrieve("http://<your ip>/snapshot.cgi?user=admin&pwd=<your password>", "mug_shot.jpg") time.sleep(2) print('Uploading image to Imgur') uploaded_image = im.upload_image(PATH, title="Uploaded with PyImgur - Mugshot") print(uploaded_image.link) print('Sending notification to device.') Client("<your imgur ID>").send_message("Mug Shot: "+ uploaded_image.link, title="Intruder Alert!") print('Notification sent!') mug_shot_ctr = mug_shot_ctr + 1 if(serial_status ==1): ser.close() print('\nProgram Ended')
Remember to change the tokens, keys, ports, the path, the camera IP, and the client ID (highlighted) to your specifics. The good thing about this code is that it checks whether the camera and Bluetooth are working as expected. If not, it gives an error message with which you can tell if something is wrong. Note that if you are not getting your camera IP to work, go back to the IP Camera Search Tool at www.netcam360.com to find the IP of your camera. It changes sometimes if it has been restarted.
What this code does is…well, everything! It first gathers all the libraries that you have used so far. It then checks and connects to the Bluetooth module followed by the IP camera. Then, it waits for Arduino to send a message (1
) saying motion has been detected. The script immediately fetches a snapshot from the IP camera's URL and uploads it to Imgur. Then, it uses Pushover to send the user an alert saying that motion has been detected, along with the Imgur URL that the user can open to see who the culprit is. The script will send three simultaneous images to give a better chance of catching the thief in action. This value can be changed by changing mug_shot_ctr
.
Power your camera and your Arduino. Let's test this out and run the program. Move your hand in front of the PIR sensor and you will get an output like this:

Immediately after the Python script has finished executing, you should see this on your smart phone:

The only thing left to do now is to position your camera in such an area where it keeps an eye on a possible entry point. The Arduino, with the PIR sensor and Bluetooth, needs to be placed close to the entry point so that the PIR sensor can detect when a door or window is opened.
It is advisable to use a 2.1mm 9-12V DC adapter to power the Arduino, as shown in the following:

Image source: http://playground.arduino.cc/Learning/WhatAdapter
Also, instead of using Eclipse, now that the code has been prepared, you can navigate to C:\<your Python project directory>
and double-click on alarm_bluetooth.py
to run it directly. It will look like this:

Done! You are finally done! You have created your very own high-tech burglar alarm system using Arduino, Bluetooth, Python, Imgur, and Pushover. If you have reached this point, I want to congratulate you. It has not been an easy journey, but it is definitely worth the patience and hard work. Your home is now secure. "However, what if we want to do this without the need for a computer?" We would have to use something such as a Raspberry Pi, but that is beyond the scope of this book. If you are adventurous, I am not going to stop you from trying to make this project standalone.
Summary
This was a long project, wasn't it? However, it is truly worth the end product, as well as the knowledge gained from each element that went together, mainly, Arduino, Bluetooth, and Python. Sometimes, instead of wasting time creating something completely from scratch, it is often a good idea to use what already exists and tweak it to do what we want. We did this for Imgur and Pushover, both very powerful tools. I hope you enjoyed and had a lot to take away from this chapter.
In the next chapter, we will take networking to a whole new level by creating a master remote for your entire home. Yes, you've guessed it – home automation.