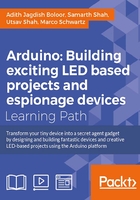
Chapter 4. Burglar Alarm – Part 1
In the previous chapter, there were a lot of coding skills to take on board. Understandably, it was difficult, but you made it through. In this chapter, we will use a lot of hardware and software. Haven't you always wanted to make your own burglar alarm? Catch the crook who stole your favorite cookies from the cookie jar? This chapter will teach you how to do just that.
Firstly, we will create a plan of how we are going to go about catching the culprit. Of course, it is not enough to simply sound an alarm when a thief is caught in the act; you also want to have evidence. You see where this is going, right? Yes, we will be using a wireless camera to get a mugshot of the culprit.
This chapter does use a fair share of code but, in addition to that, it requires quite a few components. Learning to use them in unison is the ultimate goal of this chapter. It is divided into the following sections:
- The PIR sensor
- Testing the camera
- Communicating with a smart phone
- The burglar alarm
I promised you in previous chapters that I would try to teach you as much I possibly could about the Arduino. Bluetooth is very reliable and inexpensive but it is short-range and usually needs a host to gather or send data.
The following are the components you'll need, to create a high-tech burglar alarm:
- 1 x Arduino UNO board
- 1 x USB cable A to B (aka the printer cable)
- 1 x PIR sensor
- 1 x wireless IP camera (a netcam360 is used in this chapter)
- 1 x HC-06 module (Bluetooth)
- 1 x wireless router (with accessible settings)
- 1 x PC with inbuilt Bluetooth or a Bluetooth USB module
What is a passive infrared sensor?
A passive infrared sensor (PIR) is an electronic sensor that uses infrared radiation to detect variations in its field of view. They are most commonly used as motion sensors; for example, they are used to minimize power consumption by switching off lights and utilities if nobody is at home. They are also used in state-of-the-art burglar alarm systems to trigger a switch when motion is detected.

Note
If you would like to learn more about how they work, you should refer to this page ( Arduino projects.
A mini PIR-Arduino alarm
Let's get started. We are going to create a setup in which an LED flashes when motion is detected by the PIR sensor. This is what the setup should look like when connecting the Arduino to the PIR Sensor:

Basically, the connections are as follows:
- GND → GND
- VCC → 5V
- OUT → D02 (digital pin 2)
Note
Digital pins are denoted using D and analog pins are denoted by A. So digital pin 13 is D13 and analog pin 2 is A02.
Open Arduino and load the sketch called PIR_LED.ino
, or copy this:
int ledPin = 13; // use the onboard LED int pirPin = 2; // 'out' of PIR connected to digital pin 2 int pirState = LOW; // start the state of the PIR to be low (no motion) int pirValue = 0; // variable to store change in PIR value void setup() { pinMode(ledPin, OUTPUT); // declare the LED as output pinMode(pirPin, INPUT); // declare the PIR as input Serial.begin(9600); // begin the Serial port at baud 9600 } void loop() { pirValue = digitalRead(pirPin); // read PIR value if ((pirValue == HIGH)&&(pirState==LOW)) { // check if motion has occured digitalWrite(ledPin, HIGH); // turn on LED Serial.println("Motion detected!"); pirState = HIGH; // set the PIR state to ON/HIGH delay(1000); // wait for a second } else { // if there is no motion digitalWrite(ledPin, LOW); // turn off LED if(pirState == HIGH) { // prints only if motion has happened in the first place Serial.println("No more motion!\n"); pirState = LOW; // sets the PIR state to OFF/LOW delay(200); // small delay before proceeding } } }
Run the code and open up the Serial Monitor and set the baud rate to 9600. This is a simple program that switches on the LED when motion is detected and powers it off when there is no motion.
See it in action by moving your hand in front of the PIR censor. See how the LED glows when the PIR detects motion? PIR sensors are very sensitive to variations in light, which is why they are often used in motion detectors.
This was pretty straightforward so far, right? Good! Let's now move on to the camera.
Testing the camera
An IP camera (or Internet Protocol camera) is a camera that you can access on your wireless network provided that it is configured correctly. The configuration procedure depends on which IP camera you bought and who the manufacturer is, but they should all be pretty similar.

If you purchased the exact same one that is used in this chapter, the setup is explained in the following section. However, if you got a different one, do not worry. Use the manual or installation guide that comes with the camera to install it onto your network. Go through the following steps anyway, to get an idea of what you should do if you do not have the same camera.
Installing the camera on the network
Go to download IP camera for PC.

You should also be able to find a PC installation manual on the same webpage. Follow the instructions provided. I will not go into detail because there is a high chance that you have not purchased the same IP Camera.
Eventually, you should be able to get something like this:

It is highly recommended that you create a password for your camera to keep prying eyes away. Also, do remember the password because we will need it in the steps to come.
Setting up the mugshot URL
Go back to tool or use the file of the same name located in the Useful Files
folder that comes with this chapter. I believe that this tool can be used, irrespective of the manufacturer, to find IP cameras on your network.
Run it and you should see this screen:

Note down the IP address at the top. In this case, it is 192.168.0.111:81, where 81 is the port number.
Open a new browser window and, in the address bar, paste the following:
http://<your ip>/snapshot.cgi?user=admin&pwd=<your_password>
For example:
http://192.168.0.111:81/snapshot.cgi?user=admin&pwd=password
You should be able to see a snapshot of what your camera is seeing in real time. You can try refreshing the page while moving the camera to test different camera positions. We are going to use this link later in the chapter to fetch the image file to catch the burglar.
Putting it together
As I mentioned before, we will be using a Bluetooth module to communicate with the Arduino. The specific model is the HC-06 module, which is one of the cheapest Arduino communications modules and is widely used. We could just try to hook it up to our smart phone and send an alert directly to it, but what if you aren't at home? That is a drawback of using Bluetooth.
But fret not. We will capitalize on what we can do using just the Bluetooth. I think this is a good time to explain the plan before diving into the details.
The following is a representation of what we are going to achieve in this part of the chapter.

We use the PIR sensor to check if there is any motion detected. This data is transmitted through the HC-06 Bluetooth module to a host computer through a Bluetooth channel. A script (program) running on the computer takes a snapshot of the culprit using the wireless IP camera's URL and saves it as an image. It then uploads the image to an image-sharing website and fetches the link. Finally, an alert is sent to the user saying that the alarm has been set off. The user can then open the link on a smart phone to see the culprit. I hope that wasn't too hard to follow.
First, let's get the script ready.
An introduction to Python
Wait! What? I did not sign up for this! Hold on there. We are not going to study Python too much. That is outside the scope of this book. To be honest, I think this particular section is the furthest you will deviate from the field of Arduino. But you must understand that, to create something really powerful, you need to make the most out of the resources we have. We are just going to use its basic functionalities to achieve our intended plan.
What are you even talking about? What is Python? Why are we talking about a snake? Python is a very powerful, but easy to use, language like C or Java. We will be using it to get the snapshot from the camera, upload it to the Web, and send a notification to your smart phone.
The following image is the best way to describe it:

You should be warned that this section is going to be a bit difficult, but you should try to be patient. Once Python is installed, it is going to stay there forever. Let's go ahead and install it. There are two popular versions of Python, namely 2.7 and 3.4. We are going to use 2.7 since it is older and has a lot more libraries that work with it. Yes, libraries, similar to the ones that you used earlier with the Arduino.
Download Python from https://www.python.org/download/releases/2.7.8/ according to your operating system. Note that 32-bit is x86 and 64-bit is x64.
Install it to somewhere convenient. Most OS X and Linux computers come with Python pre-installed.
Next we need to download and install an interface for writing your codes. I recommend Eclipse because it is easy to use for newcomers to Python. Since Eclipse is Java-based, you should update Java by going to http://www.java.com/en/ and installing/updating Java on your system.
You can download Eclipse from and install it, or rather extract it, into a convenient location, as with Arudino. If you have used Python before, you can simply choose your own interface. Geany is one commonly used Python IDE.
Open Eclipse. If you get a Java error, use this resource to solve the problem: http://stackoverflow.com/questions/2030434/eclipse-no-java-jre-jdk-no-virtual-machine. It will ask you for a workspace. Use something like C:\MyScripts
or choose anything similar. Then close the welcome screen. You will see something like this:

Ignore PyDev Package Explorer on the left in the screenshot. I am starting from the beginning so that you can set it up.
- Go to Help | Install New Software.
You will see this dialog box:
- Click Add….
- For Name, type
pydev
and for Location, typehttp://pydev.org/updates
. - Press OK. Wait for it to load.
- Select the first option (PyDev) and click Next >. Accept the terms and conditions and let it install. If it asks you whether you trust this application, just select Yes.
It will ask you to restart Eclipse. Allow it. Wait for it to launch by itself. You're almost done.
- Go to Window | Preferences.
In that dialog, expand PyDev | Interpreters | Python Interpreter.
- Click on New….
- For the Interpreter Name, type
Python27
.And for Interpreter Executable, you can browse to select
C:\Python27\python.exe
or you can simply paste that without quotations. Click OK.It will load a lot of files. Ensure all are checked and hit OK.
- Hit OK again.
One last thing: on the Eclipse Interface on the top right. Select PyDev instead of Java.
- Now, in PyDev Package Explorer, right-click and create a new project.
- Choose General | Project.
- Click Next >.
Call your project something like MyProject or helloworld, or whatever you like, and let it use the default location to store the files. Click Finish when you're ready.
- Right-click on MyProject | New | File.
- Call it
helloworld.py
and press Finish.Remember to use
.py
and not justhelloworld
, so that Eclipse understands what to associate it with. Type:print("Hello World!")
- Press Run (the green bubble with a right-pointing triangle). It will bring up the following window every time you run a new script:
- Simply select Python Run and press OK.
If everything works as expected, you will see this in the Console window on the right side:
Hurray! You have just written your very first Python script! You should be proud of yourself, because you have just been introduced to one of the most powerful programming tools in the history of programming. When you are done with this chapter, I recommend you look up some Python tutorials to see what it can do.
But now, we must keep moving. Let's now focus on setting up the Bluetooth network.
Hooking up the Bluetooth module
The HC-06 is a simple, low-powered Bluetooth module that works very well with Arduinos.

Go ahead and create this circuit:

The connections are as follows:
- GND → GND
- VCC → 3.3 V
- RXD → D01 (TX)
- TXD → D00 (RX)
Note that, before uploading codes where RX and TX pins are used, unplug those pins. Reconnect them once the uploading process is complete.
- Plug the Arduino into the USB hub to power the HC-06 chip. Now, in your system tray, right-click on the Bluetooth icon and click Add a Device.
- Let the computer search until it finds HC-06.
If nothing shows up, try using an Android phone to connect to it. If it doesn't show up even then, check your connections.
- Click Next.
Here, type in the device's pairing code, which is 1234 by default.
- It will now install the HC-06 on your computer. If everything works well, when you open up Device Manager and go to Ports (COM & LPT), you should see this screen:
Note down these three COM values (they will be different for different users).
- Finally, you are ready to program the Bluetooth module.
Open up a new Arduino sketch and load the ard_BL_led.ino
file or paste in the following code:
char bluetoothVal; //value sent over via bluetooth char lastValue; //stores last state of device (on/off) int ledPin = 13; void setup() { Serial.begin(9600); // begin communication on baud 9600 pinMode(ledPin, OUTPUT); // set the led pin to output } void loop() { if(Serial.available()) // searches for available data {//if there is data being recieved bluetoothVal=Serial.read(); //read it } if (bluetoothVal=='1') {//if value from bluetooth serial is '1' digitalWrite(ledPin,HIGH); // turn on LED if (lastValue!='1') Serial.println(F("LED ON")); //print LED is on lastValue = bluetoothVal; } else if (bluetoothVal=='0') {//if value from bluetooth serial is '0' digitalWrite(ledPin,LOW); //turn off LED if (lastValue!='0') Serial.println(F("LED OFF")); //print LED is off lastValue = bluetoothVal; } delay(1000); }
Again, before uploading, make sure you disconnect the RX and TX wires. Connect them after the upload is completed.
To test this code, we will use some popular software called Tera Term. OS X and Linux systems come with terminal emulators so this is not necessary. It is mainly used as a terminal emulator (a fake terminal, in plain language) to communicate with different devices/servers/ports. You can download it from http://en.osdn.jp/projects/ttssh2/releases/. Install it to someplace convenient.
Launch it and select Serial, and select the COM port that is associated with Bluetooth. Start by using the lower COM port number. If that doesn't work, the other one should.

Hit OK. Give it some time to connect. The title of the terminal window will change to COM36:9600baud if everything works correctly.
Type 1
and hit enter. What do you see? Now try 0
.

Note
Give Tera Term some time to connect to Bluetooth. 1 or 0 are not displayed when you type them. Just the LED status will be displayed.
You have now successfully controlled an LED via Bluetooth! This effect would be a lot cooler if you used a battery to power the Arduino so that there was no wired connection between the Arduino and the computer. Anyway, let's not get carried away, there is much to be done.
Before bringing everything together, there are two things left to be done: dealing with the image (mugshot) upload and sending a notification to your smart device. We'll start with the former, in the following chapter.
Summary
No, we are not done with this project. The second half of it is moved to the next chapter. But let's do a quick recap of what we have done so far. We tested out the PIR sensor, which we found out to be a really efficient motion sensor. We installed and wrote our very first Python script, which is a phenomenal achievement. Finally, we used Bluetooth to communicate between the computer and the Arduino.
In the next part of this project, we are going to process the image captured from the camera, upload it to where it can be accessible on other devices, learn about notification software, and finally bring the pieces together to create the burglar alarm.